Do you feel that your programming language is too bloated? I do, and I know I am not alone.
Let's take a look at Java. You may ask - what do you mean, when you say Java? Ah, good question. There is Java, the language, as described in
the spec. Java the Standard. Don't you wonder where's the new edition of the book, BTW? Anyway, then there's mini-edition, enterprise edition, real-time Java... there's a huge stack of official/certified technologies (e.g. all the
JEE stuff - is
JSP or
JSF Java? is
JPQL?), for which there are often multiple vendors. That's not all, there are all the popular open-source frameworks that don't bother getting Sun's approval, and yet they possess lion-share of the market (e.g. Eclipse, Spring). There's no chance to even keep track of all this, forget mastering. And yet, I don't feel that I have all I need. I have all that, and yet I can't write a descent program the way I'd like to, because I am missing some core features. What? Let's see - how about proper
modularity, closures,
tuples, local type inference, properties...
Java made the grade in expanding layer by layer - to the extent where it reminds me the state of the Earth in
Wall-E. If you haven't seen the
movie, I'll just say that the Earth drowned in human-produced garbage, and the garbage made it inhabitable for anything organic. The garbage in Java makes it impossible for the core, organic features of the language to grow. In the movie, people are leaving, and robots stay back to clean up - now you make the analogy.
As a Java programmer I really identify with Wall-E. Moving tons of garbage around, day after day, in a desperate attempt to clean up the world - something obviously impossible. And man, I'd love to be that flying-
iPod-looking Eve from outer space. She's so strong, so modern, so clean and shiny... And she has a mission!
If Wall-E is my Java, then my Eve is no doubt
Newspeak.
So what does it mean in a wider sense of programming languages? I think good language should have a small core, as little redundancy as possible. And it should grow from then on. Here's Guy Steel at OOPSLA '98:
I think that the best way of growing is via internal domain specific languages, DSLs, - you don't change the core language, yet you cover more and more domains. Anders Hejlsberg also talked about it at the recent PDC conference - adding features as a DSL; and then, if the DSL is very successful and popular, add syntactic sugar to the core language, as they did with LINQ. I don't think that you need that latter part if your language is well suited for DSLs to begin with. So the language should have a small core and be well suited for DSLs. Java isn't DSL-friendly. Scala is much better, e.g. the Actors library. Haskell, Ruby and of course Smalltalk are really good at it.
The last part is getting rid of garbage as you grow, and again,
Gilad has an
idea how to do that.
I could go on forever and ever about
DSLs, showing examples like
parser combinators, unit-test and
SQL libraries and so on. Martin Fowler
is writing a book. So, instead of boring you with repeating what's been said many times, and enumerating lots of references, I will just finish with this cutest
quote:
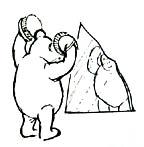
DSLs are for making languages bear-able. For I am a bear of very little brain and long words confuse me. [Milne 1926]
The premise of this subject is that computers should adapt to the ways of people, and not the other way around.